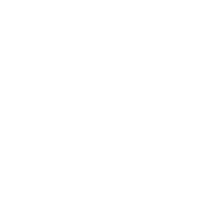
Co-authored-by: Dominik Pschenitschni <mail@celement.de> Reviewed-on: https://kolaente.dev/vikunja/frontend/pulls/2643 Co-authored-by: Dominik Pschenitschni <dpschen@noreply.kolaente.de> Co-committed-by: Dominik Pschenitschni <dpschen@noreply.kolaente.de>
35 lines
818 B
TypeScript
35 lines
818 B
TypeScript
import type {IBucket} from '@/modelTypes/IBucket'
|
|
import type {IList} from '@/modelTypes/IList'
|
|
|
|
const key = 'collapsedBuckets'
|
|
|
|
export type CollapsedBuckets = {[id: IBucket['id']]: boolean}
|
|
|
|
function getAllState() {
|
|
const saved = localStorage.getItem(key)
|
|
return saved === null
|
|
? {}
|
|
: JSON.parse(saved)
|
|
}
|
|
|
|
export const saveCollapsedBucketState = (
|
|
listId: IList['id'],
|
|
collapsedBuckets: CollapsedBuckets,
|
|
) => {
|
|
const state = getAllState()
|
|
state[listId] = collapsedBuckets
|
|
for (const bucketId in state[listId]) {
|
|
if (!state[listId][bucketId]) {
|
|
delete state[listId][bucketId]
|
|
}
|
|
}
|
|
localStorage.setItem(key, JSON.stringify(state))
|
|
}
|
|
|
|
export function getCollapsedBucketState(listId : IList['id']) {
|
|
const state = getAllState()
|
|
return typeof state[listId] !== 'undefined'
|
|
? state[listId]
|
|
: {}
|
|
}
|