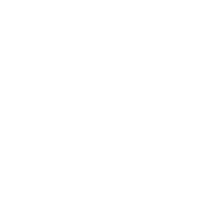
fix: project table view fix: e2e tests fix: typo in readme fix: list view route fix: don't wait until background is loaded for list to show fix: rename component imports fix: lint fix: parse task text fix: use list card grid fix: use correct class names fix: i18n keys fix: load project fix: task overview fix: list view spacing fix: find project fix: setLoading when updating a project fix: loading saved filter fix: project store loading fix: color picker import fix: cypress tests feat: migrate old list settings chore: add const for project settings fix: wrong projecten rename from lists chore: rename unused variable fix: editor list fix: shortcut list class name fix: pagination list class name fix: notifications list class name fix: list view variable name chore: clarify comment fix: i18n keys fix: router imports fix: comment chore: remove debugging leftover fix: remove duplicate variables fix: change comment fix: list view variable name fix: list view css class name fix: list item property name fix: name update tasks function correctly fix: update comment fix: project create route fix: list view class names fix: list view component name fix: result list class name fix: animation class list name fix: change debug log fix: revert a few navigation changes fix: use @ for imports of all views fix: rename link share list class fix: remove unused css class fix: dynamically import project components again
86 lines
2.0 KiB
Vue
86 lines
2.0 KiB
Vue
<template>
|
|
<Multiselect
|
|
class="control is-expanded"
|
|
:placeholder="$t('project.search')"
|
|
:search-results="foundProjects"
|
|
label="title"
|
|
:select-placeholder="$t('project.searchSelect')"
|
|
:model-value="project"
|
|
@update:model-value="Object.assign(project, $event)"
|
|
@select="select"
|
|
@search="findProjects"
|
|
>
|
|
<template #searchResult="{option}">
|
|
<span class="project-namespace-title search-result">{{ namespace((option as IProject).namespaceId) }} ></span>
|
|
{{ (option as IProject).title }}
|
|
</template>
|
|
</Multiselect>
|
|
</template>
|
|
|
|
<script lang="ts" setup>
|
|
import {reactive, ref, watch} from 'vue'
|
|
import type {PropType} from 'vue'
|
|
import {useI18n} from 'vue-i18n'
|
|
|
|
import type {IProject} from '@/modelTypes/IProject'
|
|
import type {INamespace} from '@/modelTypes/INamespace'
|
|
|
|
import {useProjectStore} from '@/stores/projects'
|
|
import {useNamespaceStore} from '@/stores/namespaces'
|
|
|
|
import ProjectModel from '@/models/project'
|
|
|
|
import Multiselect from '@/components/input/multiselect.vue'
|
|
|
|
const props = defineProps({
|
|
modelValue: {
|
|
type: Object as PropType<IProject>,
|
|
required: false,
|
|
},
|
|
})
|
|
const emit = defineEmits(['update:modelValue'])
|
|
|
|
const {t} = useI18n({useScope: 'global'})
|
|
|
|
const project: IProject = reactive(new ProjectModel())
|
|
|
|
watch(
|
|
() => props.modelValue,
|
|
(newProject) => Object.assign(project, newProject),
|
|
{
|
|
immediate: true,
|
|
deep: true,
|
|
},
|
|
)
|
|
|
|
const projectStore = useProjectStore()
|
|
const namespaceStore = useNamespaceStore()
|
|
const foundProjects = ref<IProject[]>([])
|
|
function findProjects(query: string) {
|
|
if (query === '') {
|
|
select(null)
|
|
}
|
|
foundProjects.value = projectStore.searchProject(query)
|
|
}
|
|
|
|
function select(l: IProject | null) {
|
|
if (l === null) {
|
|
return
|
|
}
|
|
Object.assign(project, l)
|
|
emit('update:modelValue', project)
|
|
}
|
|
|
|
function namespace(namespaceId: INamespace['id']) {
|
|
const namespace = namespaceStore.getNamespaceById(namespaceId)
|
|
return namespace !== null
|
|
? namespace.title
|
|
: t('project.shared')
|
|
}
|
|
</script>
|
|
|
|
<style lang="scss" scoped>
|
|
.project-namespace-title {
|
|
color: var(--grey-500);
|
|
}
|
|
</style> |