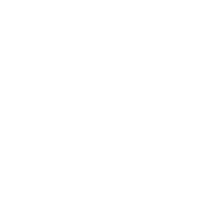
fix: project table view fix: e2e tests fix: typo in readme fix: list view route fix: don't wait until background is loaded for list to show fix: rename component imports fix: lint fix: parse task text fix: use list card grid fix: use correct class names fix: i18n keys fix: load project fix: task overview fix: list view spacing fix: find project fix: setLoading when updating a project fix: loading saved filter fix: project store loading fix: color picker import fix: cypress tests feat: migrate old list settings chore: add const for project settings fix: wrong projecten rename from lists chore: rename unused variable fix: editor list fix: shortcut list class name fix: pagination list class name fix: notifications list class name fix: list view variable name chore: clarify comment fix: i18n keys fix: router imports fix: comment chore: remove debugging leftover fix: remove duplicate variables fix: change comment fix: list view variable name fix: list view css class name fix: list item property name fix: name update tasks function correctly fix: update comment fix: project create route fix: list view class names fix: list view component name fix: result list class name fix: animation class list name fix: change debug log fix: revert a few navigation changes fix: use @ for imports of all views fix: rename link share list class fix: remove unused css class fix: dynamically import project components again
110 lines
2.8 KiB
TypeScript
110 lines
2.8 KiB
TypeScript
import {createFakeUserAndLogin} from '../../support/authenticateUser'
|
|
|
|
import {UserProjectFactory} from '../../factories/users_project'
|
|
import {TaskFactory} from '../../factories/task'
|
|
import {UserFactory} from '../../factories/user'
|
|
import {ProjectFactory} from '../../factories/project'
|
|
import {prepareProjects} from './prepareProjects'
|
|
|
|
describe('Project View Project', () => {
|
|
createFakeUserAndLogin()
|
|
prepareProjects()
|
|
|
|
it('Should be an empty project', () => {
|
|
cy.visit('/projects/1')
|
|
cy.url()
|
|
.should('contain', '/projects/1/list')
|
|
cy.get('.project-title')
|
|
.should('contain', 'First Project')
|
|
cy.get('.project-title-dropdown')
|
|
.should('exist')
|
|
cy.get('p')
|
|
.contains('This project is currently empty.')
|
|
.should('exist')
|
|
})
|
|
|
|
it('Should create a new task', () => {
|
|
const newTaskTitle = 'New task'
|
|
|
|
cy.visit('/projects/1')
|
|
cy.get('.task-add textarea')
|
|
.type(newTaskTitle+'{enter}')
|
|
cy.get('.tasks')
|
|
.should('contain.text', newTaskTitle)
|
|
})
|
|
|
|
it('Should navigate to the task when the title is clicked', () => {
|
|
const tasks = TaskFactory.create(5, {
|
|
id: '{increment}',
|
|
project_id: 1,
|
|
})
|
|
cy.visit('/projects/1/list')
|
|
|
|
cy.get('.tasks .task .tasktext')
|
|
.contains(tasks[0].title)
|
|
.first()
|
|
.click()
|
|
|
|
cy.url()
|
|
.should('contain', `/tasks/${tasks[0].id}`)
|
|
})
|
|
|
|
it('Should not see any elements for a project which is shared read only', () => {
|
|
UserFactory.create(2)
|
|
UserProjectFactory.create(1, {
|
|
project_id: 2,
|
|
user_id: 1,
|
|
right: 0,
|
|
})
|
|
const projects = ProjectFactory.create(2, {
|
|
owner_id: '{increment}',
|
|
namespace_id: '{increment}',
|
|
})
|
|
cy.visit(`/projects/${projects[1].id}/`)
|
|
|
|
cy.get('.project-title-wrapper .icon')
|
|
.should('not.exist')
|
|
cy.get('input.input[placeholder="Add a new task..."')
|
|
.should('not.exist')
|
|
})
|
|
|
|
it('Should only show the color of a project in the navigation and not in the project view', () => {
|
|
const projects = ProjectFactory.create(1, {
|
|
hex_color: '00db60',
|
|
})
|
|
TaskFactory.create(10, {
|
|
project_id: projects[0].id,
|
|
})
|
|
cy.visit(`/projects/${projects[0].id}/`)
|
|
|
|
cy.get('.menu-list li .list-menu-link .color-bubble')
|
|
.should('have.css', 'background-color', 'rgb(0, 219, 96)')
|
|
cy.get('.tasks .color-bubble')
|
|
.should('not.exist')
|
|
})
|
|
|
|
it('Should paginate for > 50 tasks', () => {
|
|
const tasks = TaskFactory.create(100, {
|
|
id: '{increment}',
|
|
title: i => `task${i}`,
|
|
project_id: 1,
|
|
})
|
|
cy.visit('/projects/1/list')
|
|
|
|
cy.get('.tasks')
|
|
.should('contain', tasks[1].title)
|
|
cy.get('.tasks')
|
|
.should('not.contain', tasks[99].title)
|
|
|
|
cy.get('.card-content .pagination .pagination-link')
|
|
.contains('2')
|
|
.click()
|
|
|
|
cy.url()
|
|
.should('contain', '?page=2')
|
|
cy.get('.tasks')
|
|
.should('contain', tasks[99].title)
|
|
cy.get('.tasks')
|
|
.should('not.contain', tasks[1].title)
|
|
})
|
|
}) |