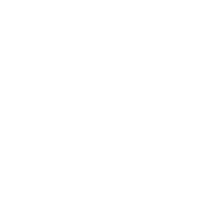
fix: project table view fix: e2e tests fix: typo in readme fix: list view route fix: don't wait until background is loaded for list to show fix: rename component imports fix: lint fix: parse task text fix: use list card grid fix: use correct class names fix: i18n keys fix: load project fix: task overview fix: list view spacing fix: find project fix: setLoading when updating a project fix: loading saved filter fix: project store loading fix: color picker import fix: cypress tests feat: migrate old list settings chore: add const for project settings fix: wrong projecten rename from lists chore: rename unused variable fix: editor list fix: shortcut list class name fix: pagination list class name fix: notifications list class name fix: list view variable name chore: clarify comment fix: i18n keys fix: router imports fix: comment chore: remove debugging leftover fix: remove duplicate variables fix: change comment fix: list view variable name fix: list view css class name fix: list item property name fix: name update tasks function correctly fix: update comment fix: project create route fix: list view class names fix: list view component name fix: result list class name fix: animation class list name fix: change debug log fix: revert a few navigation changes fix: use @ for imports of all views fix: rename link share list class fix: remove unused css class fix: dynamically import project components again
63 lines
1.8 KiB
TypeScript
63 lines
1.8 KiB
TypeScript
import type { IProject } from '@/modelTypes/IProject'
|
|
|
|
type ProjectView = Record<IProject['id'], string>
|
|
|
|
const DEFAULT_PROJECT_VIEW = 'project.list' as const
|
|
const PROJECT_VIEW_SETTINGS_KEY = 'projectView'
|
|
|
|
/**
|
|
* Save the current project view to local storage
|
|
*/
|
|
export function saveProjectView(projectId: IProject['id'], routeName: string) {
|
|
if (routeName.includes('settings.')) {
|
|
return
|
|
}
|
|
|
|
if (!projectId) {
|
|
return
|
|
}
|
|
|
|
// We use local storage and not the store here to make it persistent across reloads.
|
|
const savedProjectView = localStorage.getItem(PROJECT_VIEW_SETTINGS_KEY)
|
|
let savedProjectViewJson: ProjectView | false = false
|
|
if (savedProjectView !== null) {
|
|
savedProjectViewJson = JSON.parse(savedProjectView) as ProjectView
|
|
}
|
|
|
|
let projectView: ProjectView = {}
|
|
if (savedProjectViewJson) {
|
|
projectView = savedProjectViewJson
|
|
}
|
|
|
|
projectView[projectId] = routeName
|
|
localStorage.setItem(PROJECT_VIEW_SETTINGS_KEY, JSON.stringify(projectView))
|
|
}
|
|
|
|
export const getProjectView = (projectId: IProject['id']) => {
|
|
// Migrate old setting over
|
|
// TODO: remove when 1.0 release
|
|
const oldListViewSettings = localStorage.getItem('listView')
|
|
if (oldListViewSettings !== null) {
|
|
localStorage.setItem(PROJECT_VIEW_SETTINGS_KEY, oldListViewSettings)
|
|
localStorage.removeItem('listView')
|
|
}
|
|
|
|
// Remove old stored settings
|
|
// TODO: remove when 1.0 release
|
|
const savedProjectView = localStorage.getItem(PROJECT_VIEW_SETTINGS_KEY)
|
|
if (savedProjectView !== null && savedProjectView.startsWith('project.')) {
|
|
localStorage.removeItem(PROJECT_VIEW_SETTINGS_KEY)
|
|
}
|
|
|
|
if (!savedProjectView) {
|
|
return DEFAULT_PROJECT_VIEW
|
|
}
|
|
|
|
const savedProjectViewJson: ProjectView = JSON.parse(savedProjectView)
|
|
|
|
if (!savedProjectViewJson[projectId]) {
|
|
return DEFAULT_PROJECT_VIEW
|
|
}
|
|
|
|
return savedProjectViewJson[projectId]
|
|
} |